A few weeks ago, Angular 18 was officially released, and we have already had the opportunity to try it out and explore its improvements. Angular 18 is the latest version of this popular framework, which comes with a series of new features and optimizations that promise to further enhance web application development. In this article, we will tell you about its main new features and how they can benefit developers.
1. What’s new in Angular 18
2. Zoneless Change detection (Experimental Support)
– Implementing Zoneless
– Default Event Grouping in Angular 18
– Zoneless and Native Await
3. Signals
4. New Features in Forms
– State and Value Change Events
5. Deferrable views
6. New Control Flow Directives
7. Ng-content with Alternative Content
8. Event replay
-What is “Event Replay”?
9. Material 3 is Stable
10. angular.dev The New Official Angular Website
angular.dev The New Official Angular Website
Without a doubt, and after reviewing this new version, we see that Angular 18 has focused on performance improvement and simplifying development.
Zoneless Change detection (Soporte experimental)
n previous versions, Angular uses the ZoneJS library to trigger change detection. This dependency has certain disadvantages in terms of performance and development experience.
With the new version 18, Angular aims to start removing this dependency and has included its own change detection. This new Zoneless functionality eliminates the dependency on Zone.js, but it is important to note that this functionality is experimental, so it is still in development and not recommended for production use.
Implementing Zoneless
To try Zoneless in our projects, we just need to add provideExperimentalZonelessChangeDetection
in our bootstrap.
bootstrapApplication(App, { providers: [ provideExperimentalZonelessChangeDetection() ] });
After this change, you can remove ZoneJS from your polyfills in the angular.json file, as long as you don’t have dependencies that affect this library.
Developers should gradually adapt to these changes because in the future we will have:
- Greater compatibility for micro frontends and interoperability with other frameworks
- Much faster rendering and execution times
- Easier code debugging
- Much lighter websites and applications, allowing for improved loading speeds.
The best way to use zoneless is with the use of Signals.
@Component({
...
template: `
<h1>Hello from {{ name() }}!</h1>
<button (click)="handleClick()">Go Zoneless</button>
`,
})
export class App {
protected name = signal('Angular');
handleClick() {
this.name.set('Zoneless Angular');
}
}
In the previous example, clicking the button invokes the handleClick
method, which updates the signal value and the user interface. This works similarly to an application using zone.js, with some key differences. With zone.js, Angular executed change detection whenever the application state might have changed. Without zones, Angular limits this check to fewer triggers, such as signal updates.
This change includes a new scheduler that avoids multiple checks. For example, when the user clicks the button, Angular will execute change detection only once. For more information, you can consult the official documentation.
Default Event Grouping in Angular 18
Starting with Angular 18, the same scheduler is used for zoneless applications and for applications using zone.js. This is to reduce the number of change detection cycles in new zone.js applications.
This behavior is only enabled for new applications, as it may cause errors in applications that rely on the previous change detection behavior.
To support both event technologies in existing projects, configure your NgZone provider in bootstrapApplication
:
import { bootstrapApplication, provideZoneChangeDetectionCoalescing } from '@angular/core'; bootstrapApplication(AppComponent, { providers: [provideZoneChangeDetectionCoalescing(true)] });
Zoneless and Native Await
Now, when creating an application with zoneless change detection, Angular CLI uses native async/await without converting it to promises. This improves debugging and reduces package sizes.
With ZoneJS, this was not the case. With this library, every time async/await was used in your application, it was transformed into promises by Angular CLI.
Signals
Signals, introduced in previous versions of Angular, have been optimized in Angular 18 to offer better performance. They allow more efficient communication between components, reducing response time and improving user experience.
Key Benefits:
- Better Performance: Optimization in the update and management of reactive data.
- Efficiency: Lower latency in communication between components.
- User Experience: Faster and smoother responses in web applications.
import { signal } from 'angular/signals'; const count = signal(0); // Optimized signal usage count.set(1); console.log(count.value); // Outputs: 1
New Features in Forms
Angular 18 introduces new features in form management, offering greater robustness and flexibility in handling states and values.
State and Value Change Events
It is now possible to track changes in the state and value of forms through unified events. This allows for greater reactivity and control over forms, improving validation and dynamic updates.
These improvements simplify the management of complex forms, making it easier to create more efficient applications.
const nameControl = new FormControl<string|null>('name', Validators.required); nameControl.events.subscribe(event => { // process the individual events });
Deferrable views
@defer is now stable, allowing you to use templates or components deferred when a condition is met. You can find everything about deferrable views in the official documentation.
New Control Flow Directives
Angular 18 introduces new directives:
- @if
- @else
@if (a > b) { {{a}} is greater than {{b}} } @else if (b > a) { {{a}} is less than {{b}} } @else { {{a}} is equal to {{b}} }
@switch
@switch (condition) { @case (caseA) { Case A. } @case (caseB) { Case B. } @default { Default case. } }
In this way, the new directives simplify the use of *ngIf and *ngSwitch, allowing for cleaner code. These are just a few examples; you can find all the details in the official control-flow documentation.
Ng-content with Alternative Content
We can now add alternative content within ng-content
, and it will be displayed if no content is projected into the component.
@Component({
selector: 'app-profile',
template: `
<ng-content select=".greeting">Hello </ng-content>
<ng-content>Unknown user</ng-content>
`,
})
export class Profile {}
Now, by adding select="name"
, you can define the alternative content. For example, in this component:
<app-profile>
<span class="greeting">Good morning </span>
</app-profile>
The result would be as follows:
<span class="greeting">Good morning </span>
Unknown user
Event replay
A few months ago, Angular announced that they would start working on merging Angular with an internal framework used by Google called Wiz to improve performance. Thanks to this, Angular is incorporating more features focused on this improvement, such as partial hydration. To achieve this, Angular has included event dispatch in its monorepo, enhancing the event replay function in hybrid rendering.
What is “Event Replay”?
“Event replay” records user events while the page is loading and replays them once the application has loaded, ensuring that user actions are not lost. This is useful on slow connections, where user events are processed once the page is fully loaded.
For example, if an e-commerce website takes a long time to load, or if the user has a poor connection, the user might click the add-to-cart button several times before the page has fully loaded. In previous versions, without event replay, these actions would be lost.
But with the new version of Angular, these actions would be recorded even before the page finishes loading, and once the loading is complete, these actions would be replayed, adding as many products to the cart as the user clicked.
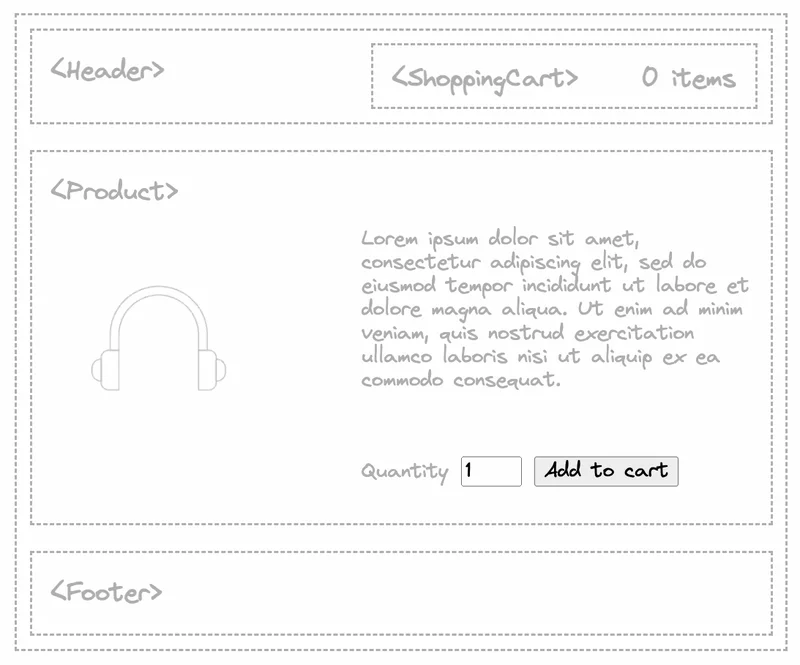
Event replay angular18
The event replay feature is available in Angular 18 in developer preview. You can enable it using withEventReplay()
.
bootstrapApplication(App, { providers: [ provideClientHydration(withEventReplay()) ] });
Material 3 is Stable
Angular 18 introduces Material 3 with a series of innovations that enhance user experience and optimize application development.
Adaptive Design
More flexible and responsive interfaces, better suited to multiple devices and screen sizes.
New Components
Incorporation of new components and improvements to existing ones, providing more options and functionalities.
Modernized Themes
Updated visual themes with a modern aesthetic and advanced customization options.
Accessibility Improvements
Focus on accessibility, improving contrast, navigation, and support for assistive technologies.
Enhanced Compatibility
Better integration with the latest versions of Angular and ecosystem tools.
Expanded Documentation
Updated documentation with practical examples and detailed guides to facilitate the adoption of Material 3.
angular.dev The new official Angular website.
And finally, Angular launched a much more modern website, https://angular.dev/, where you can find all the official documentation and practical tutorials based on WebContainers.
You can find more content on our blog.